WebAssembly vs JavaScript: A Head-to-head Comparison
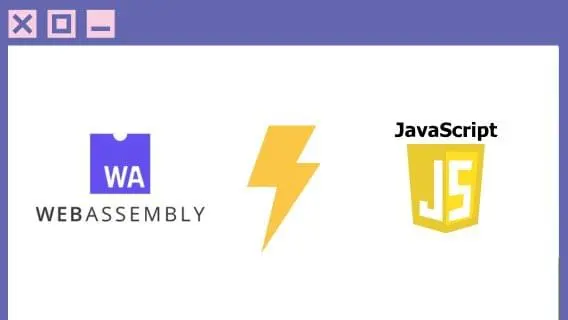
Performance
WebAssembly (Wasm) typically outperforms JavaScript for specific tasks due to its binary instruction format, which allows for near-native speed execution in modern web browsers. The low-level nature of Wasm enables efficient execution by browser engines, making it particularly advantageous for performance-intensive tasks like numerical calculations, data processing, and game rendering. Despite its speed benefits, Wasm is designed to complement rather than replace JavaScript. JavaScript remains the dominant language for web development because of its ease of use, flexibility, and extensive ecosystem, while WebAssembly is leveraged for tasks that require enhanced performance.
Portability and Compatibility
Both Wasm and JavaScript are highly portable, working across all major browsers and platforms.
Wasm has a unique advantage: it supports multiple programming languages. You can write code in C, C++, Rust, and other languages, compile it to Wasm, and run it on the web. This opens the door for more developers and allows code reuse from other environments.
On the other hand, JavaScript enjoys universal support and boasts a massive ecosystem of frameworks and libraries, making it the default for most web projects.
Ease of Use
JavaScript’s low learning curve and dynamic nature make it beginner-friendly. With extensive documentation and a vibrant community, JavaScript is accessible and productive. Tools and frameworks abound, simplifying development.
WebAssembly, while powerful, is more complex. It requires knowledge of languages like C++ or Rust and an understanding of the compilation process. Its ecosystem is also still maturing, offering fewer resources than JavaScript.
Security
Both Wasm and JavaScript run in sandboxed environments, safeguarding the host system. However, JavaScript’s dynamic nature can lead to vulnerabilities like cross-site scripting (XSS) if not handled properly. Wasm’s binary format and structure make it more resistant to certain attacks, such as code injection. But as with any technology, best practices are essential to ensure security.
Community and Ecosystem
JavaScript’s community is enormous, with millions of developers and a mature ecosystem of libraries, frameworks, and tools. Resources like Stack Overflow, GitHub, and numerous online courses provide ample support.
WebAssembly’s community, while smaller, is growing fast. Organizations like the Bytecode Alliance are expanding the ecosystem with new tools and libraries, and as more companies adopt Wasm for high-performance applications, this trend is expected to continue.
What’s Trending with WebAssembly and JavaScript
Adoption and Usage
WebAssembly is gaining significant traction in 2024, witnessing a 23% increase in adoption compared to the previous year. Industries such as gaming, finance, and healthcare are leveraging Wasm to develop high-performance web applications that demand real-time processing capabilities.
In contrast, JavaScript remains the dominant force in web development, with more than 60% of developers regularly utilizing it. Its flexibility and vast ecosystem render it essential for a diverse array of applications.
Innovations and Updates
This year has been transformative for WebAssembly. The introduction of the WebAssembly System Interface (WASI) has streamlined the execution of Wasm outside the browser, paving the way for new applications, including server-side solutions and Internet of Things (IoT) devices. Additionally, enhancements to the WebAssembly component model have improved the efficiency of modularization and reuse of Wasm modules.
JavaScript is also advancing with new proposals under ECMAScript. In 2024, features such as enhanced pattern matching, improved asynchronous programming capabilities, and better modularity have been officially standardized. These enhancements aim to bolster JavaScript’s robustness and versatility, addressing frequent challenges faced by developers.
Tooling and Frameworks
The tooling ecosystem for WebAssembly has progressed significantly. Projects like Wasmtime and Wasmer have made it easier to execute Wasm on servers, while tools such as wasm-pack facilitate the integration of Wasm into web applications. The Bytecode Alliance is actively working on new libraries and tools to enhance the Wasm development experience.
Meanwhile, JavaScript frameworks like React, Vue, and Angular are continuously improving, with updates focused on performance, enhancing the developer experience, and integrating modern web standards. The emergence of new frameworks and tools further highlights the dynamic and evolving nature of the JavaScript ecosystem.
Success Stories in Practice
Numerous companies are experiencing significant advantages from adopting WebAssembly. For instance, AutoDesk has implemented Wasm to enhance the efficiency of its CAD software, resulting in a swifter and more responsive user experience. Additionally, financial institutions are utilizing Wasm for intricate real-time calculations, which improves the speed and precision of their trading systems.
Meanwhile, JavaScript continues to dominate the web development landscape. Firms like Airbnb and Netflix depend on JavaScript to create seamless, interactive front-end experiences. The adaptability of JavaScript enables these companies to rapidly iterate and roll out new features, ensuring they stay ahead of the competition.
Example Code
WebAssembly example: A simple addition function
This example shows how to use WebAssembly with Rust and JavaScript to perform a simple addition of two numbers.
1.Rust Code (src/lib.rs)
// src/lib.rs
#[no_mangle]
pub extern "C" fn add(a: i32, b: i32) -> i32 {
a + b
}
Explanation in simple terms:
#[no_mangle]
: This is a special instruction for the Rust compiler. It tells the compiler not to change the name of the function, so it can be easily called from other programming languages or environments.pub extern "C" fn add(a: i32, b: i32) -> i32
: This line defines a public function namedadd
that can be used in C-style programming languages. It takes two inputs, both of which are 32-bit integers (calledi32
in Rust), and it returns their sum as another 32-bit integer.
2. Compiling to WebAssembly
rustup target add wasm32-unknown-unknown
cargo build --target wasm32-unknown-unknown --release
rustup target add wasm32-unknown-unknown
: This command adds support for the WebAssembly target to your Rust toolchain. It allows you to compile your Rust code into WebAssembly format.cargo build --target wasm32-unknown-unknown --release
: This command compiles your Rust code into WebAssembly. The--release
flag tells the compiler to optimize the code for performance, generating a.wasm
file that you can use in your web applications.
3.JavaScript integration
async function loadWasm() {
const response = await fetch('path/to/your.wasm');
const buffer = await response.arrayBuffer();
const { instance } = await WebAssembly.instantiate(buffer);
console.log(instance.exports.add(2, 3)); // Output: 5
}
loadWasm();
Explanation in Simple Terms:
Fetching the WebAssembly File: This segment of the code retrieves the .wasm file generated from the web server.
Using the WebAssembly Function:
response.arrayBuffer()
: This prepares the WebAssembly file for utilization.WebAssembly.instantiate(buffer)
: This loads the WebAssembly file, enabling us to access its functions.instance.exports.add(2, 3)
: This invokes theadd
function from the WebAssembly file with the arguments 2 and 3, outputting the result (5).
Where to Place This Code:
- Rust Code: Save this in a file named
lib.rs
located in thesrc
folder of your Rust project. - Compiling Command: Execute the compilation commands in your terminal.
- JavaScript Code: Save this in a
.js
file, such asloadWasm.js
, and ensure it is linked in your HTML file.
JavaScript example: Fetching data from an API
This example demonstrates how to use JavaScript to fetch data from an online service (API) and display it on a web page.
HTML (index.html):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fetch API Example</title>
</head>
<body>
<div id="data"></div>
<script src="app.JavaScript"></script>
</body>
</html>
JavaScript (app.JavaScript):
async function fetchData() {
const response = await fetch('https://api.example.com/data');
const data = await response.JavaScripton();
document.getElementById('data').innerText = JavaScriptON.stringify(data, null, 2);
}
fetchData();
Explanation in Simple Terms:
HTML Structure: The HTML file creates a basic webpage that includes a section (<div id="data"></div>
) where the fetched data will be displayed. It also links to the JavaScript file (app.js
).
Fetching Data with JavaScript:
fetch('https://api.example.com/data')
: This line instructs the browser to send a request for data from a specified online source (API).await response.json()
: This converts the incoming data into a format (JSON) that is easier to work with.document.getElementById('data').innerText = JSON.stringify(data, null, 2)
: This line displays the fetched data on the webpage in a neatly formatted manner.
Where to Place This Code:
- HTML File: Save this code in a file named
index.html
. - JavaScript File: Place the JavaScript code in a file named
app.js
, ensuring it is linked correctly in the HTML file as shown.
Summary
- WebAssembly Example: This demonstrates how to add two numbers using Rust and WebAssembly, then utilize this function within a webpage through JavaScript.
- JavaScript Example: This showcases how to retrieve data from an API and present it on a webpage.
Both examples highlight the integration of robust functionalities into your web projects by employing contemporary web development methods.
WebAssembly vs. JavaScript: Pros and Cons
Advantages of WebAssembly
Incredible Performance
One of the standout features of WebAssembly is its remarkable speed. It operates at near-native execution speeds, making it particularly suited for tasks that require significant computational power, such as gaming, 3D rendering, image and video processing, and scientific simulations. This performance enhancement is attributed to several key factors:
- Ahead-of-time (AOT) Compilation: WebAssembly code is compiled before it is loaded into the browser, which leads to quicker execution compared to JavaScript’s just-in-time (JIT) compilation.
- Static Typing: The static typing system in WebAssembly facilitates aggressive optimizations during the compilation process, which contributes to improved performance.
- Direct Hardware Access: WebAssembly provides a low-level virtual machine, allowing developers to access hardware features such as SIMD (Single Instruction, Multiple Data) instructions, enabling the utilization of modern processor capabilities.
Support for Multiple Languages
WebAssembly is not confined to just one programming language; it supports various languages, including C, C++, Rust, Go, and more. This versatility allows developers to utilize their existing knowledge and select the language that aligns best with their project needs.
Improved Security
WebAssembly operates within a sandboxed environment, offering a greater level of security compared to JavaScript. This isolation minimizes the risk of malicious code accessing sensitive information or affecting system resources.
Disadvantages of WebAssembly
- Limited EcosystemDespite its increasing adoption, WebAssembly’s ecosystem remains relatively immature compared to JavaScript. There are fewer libraries, frameworks, and tools specifically tailored for WebAssembly, which may necessitate extra effort from developers to find suitable resources and support.
- Steeper Learning CurveWorking with WebAssembly often requires familiarity with low-level programming concepts, such as memory management and compilation processes. This can pose challenges for developers who are more accustomed to working with high-level languages, making the transition to WebAssembly less straightforward.
- Browser Compatibility IssuesAlthough major browsers provide support for WebAssembly, there can be minor differences in implementation and available features across various browsers. This inconsistency may require additional testing and adjustments to ensure functionality across all platforms.
Advantages of JavaScript
JavaScript serves as the cornerstone of interactive web experiences, powering everything from simple animations to complex single-page applications. Its widespread use, extensive ecosystem, and user-friendly nature have established it as the most popular programming language for web development. Here are some of its key benefits:
- Ubiquitous and VersatileJavaScript is supported by nearly every web browser, making it the most accessible language for web development. It is not only utilized for frontend development but also plays a crucial role in server-side development (with Node.js), mobile applications (such as those built with React Native), and desktop applications (using frameworks like Electron).
- Rich EcosystemThe JavaScript ecosystem is incredibly diverse, boasting a vast array of libraries (like React, Vue, and Angular), frameworks, and tools for nearly every conceivable task. This wealth of resources streamlines the development process, enabling developers to construct complex applications with efficiency.
- Ease of LearningJavaScript’s syntax is relatively forgiving and straightforward, making it an excellent choice for beginners. The extensive community support and abundant online tutorials further ease the learning journey, helping new developers to get up to speed quickly.
Cons of JavaScript
- Performance Bottlenecks
Despite the advancements in JavaScript engines, the language can still struggle with performance when handling heavy computational tasks. This is a domain where WebAssembly excels, offering faster execution speeds for demanding operations. - Single-Threaded Nature
JavaScript operates on a single-threaded model, allowing only one task to run at a time. Although features like Web Workers and asynchronous programming help alleviate this constraint, it can still create challenges for applications that require concurrent processing.
Choosing the Right Tool
Selecting between WebAssembly (Wasm) and JavaScript hinges on the specific requirements of your project. If your focus is on achieving exceptional performance for demanding tasks like gaming or intricate simulations, Wasm is your ideal choice. Conversely, if you value ease of use, widespread compatibility, and access to a rich ecosystem, JavaScript is the recommended option.
Future Outlook
The prospects for WebAssembly are promising, with rising adoption rates and a burgeoning ecosystem. As more tools and libraries emerge, Wasm will become increasingly accessible for developers. Its applications extend beyond the browser, with potential uses in server-side development and IoT devices.
JavaScript, on the other hand, is set to maintain its leading position in web development. Continuous standardization and a vibrant community will ensure that JavaScript evolves to meet new challenges. As web applications grow more complex, its flexibility and extensive resources will remain essential.
Conclusion
Both WebAssembly and JavaScript possess unique strengths and play vital roles in contemporary web development. Wasm is particularly suited for scenarios where performance is critical, while JavaScript is crucial for creating interactive and engaging web applications. By understanding their distinctions and keeping up with the latest trends, developers can make well-informed choices and utilize the most effective tools for their projects.
As technology progresses, it is important for developers to engage with both WebAssembly and JavaScript. Staying informed about these technologies will better prepare you to face the demands of modern web development. Whether you’re developing high-performance applications with Wasm or crafting dynamic user interfaces with JavaScript, the future of web development is filled with exciting opportunities.