Even when images are optimized effectively, they can still be quite large, causing delays for users trying to access content on your website. Many users may become impatient and leave for another site unless you implement a solution for image loading that enhances the perceived speed.
In this article, you will discover five methods for lazy loading images that can be added to your web optimization toolkit, ultimately enhancing the user experience on your site. These techniques can be applied to lazy load different types of images in your application, including background images, inline images, and banner images.
Key Takeaways
Quicker Page Loads: Implementing lazy loading for images boosts your website’s speed by only loading images that are currently visible in the user’s viewport.Enhanced User Experience: Lazy loading improves the browsing experience, particularly for users on slower connections or mobile devices, by minimizing load times.Bandwidth Efficiency: Images are loaded only when necessary, conserving bandwidth and lowering data consumption for both users and servers.Easy Implementation: Lazy loading can be easily integrated using the HTML “loading” attribute or more advanced techniques such as the Intersection Observer API.SEO Advantages: Improved load times from lazy loading can positively impact search engine rankings, enhancing your site’s performance in search results.
What Is Lazy Loading?
Lazy loading images refers to the asynchronous loading of images on websites. This technique allows content to load only when the user scrolls, ensuring that above-the-fold content is fully loaded first, and additional images appear only when they enter the browser’s viewport. Consequently, if users do not scroll down, images located at the bottom of the page are not loaded, leading to enhanced application performance.
For web developers, understanding how to implement lazy loading in HTML is crucial, as many websites utilize this method. For example, when you browse your favorite site for high-resolution photos, you may notice that only a limited number of images load initially. As you scroll down the page, placeholder images are quickly replaced with the actual images for preview.
A great illustration of this is the loader on Unsplash.com: when a portion of the page is scrolled into view, a placeholder is replaced with a high-resolution photo.
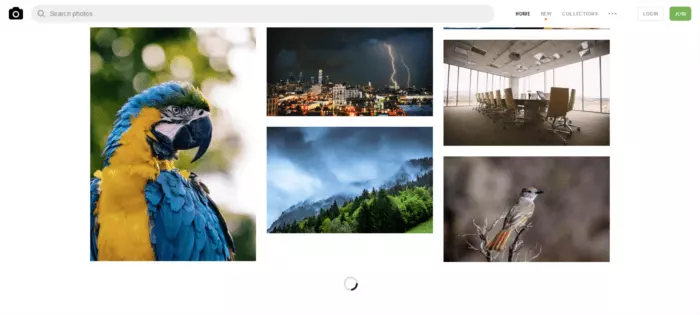
Why You Should Implement Lazy Loading for Images
Understanding how to lazy load images is essential for optimizing web performance, particularly on pages with heavy visual content. Here are a few compelling reasons to consider lazy loading images for your website:
Enhances DOM Loading TimeIf your website relies on JavaScript to display content or provide user functionality, quickly loading the DOM is crucial. Often, scripts are designed to execute only after the DOM has fully loaded. On a site featuring numerous images, implementing lazy loading—loading images asynchronously—can significantly influence whether users stay engaged or leave your website.Reduces Bandwidth ConsumptionMost lazy loading solutions function by only loading images when users scroll to the point where they become visible in the viewport. This means that images will not be loaded if users do not reach that section, resulting in substantial bandwidth savings. This is particularly beneficial for users accessing the web on mobile devices or slow connections, who will certainly appreciate this efficiency.
1. Native Lazy Loading
Native lazy loading for images and iframes is an uncomplicated approach that allows you to lazy load content as users scroll through a webpage. To implement this, simply add the loading="lazy"
attribute to your images and iframes:
<img src="myimage.jpg" loading="lazy" alt="..." />
<iframe src="content.html" loading="lazy"></iframe>
As demonstrated, there’s no need for JavaScript or dynamic swapping of the src
attribute; it’s just simple HTML. This method exemplifies how to incorporate lazy loading in HTML without any additional complexity.
The loading
attribute allows you to delay loading off-screen images and iframes until users scroll to their position on the page. It can take one of three values:
- lazy: Ideal for lazy loading.
- eager: Instructs the browser to load the specified content immediately.
- auto: Leaves the decision of whether to lazy load or not up to the browser.
This method stands out for its minimal overhead and its clean, straightforward implementation of lazy loading images in HTML. However, while most major browsers provide good support for the loading
attribute, some still lack full compatibility as of this writing.
For a comprehensive article on this excellent feature for HTML lazy loading, including workarounds for browser support, be sure to check out Addy Osmani’s “Native image lazy-loading for the web!”
2. Lazy Loading Using the Intersection Observer API
The Intersection Observer API is a contemporary interface you can use for lazy loading images and other types of content.As described by MDN, the Intersection Observer API allows you to asynchronously observe changes in the intersection of a target element with an ancestor element or the top-level document’s viewport.
In simpler terms, this API monitors how one element intersects with another asynchronously.
Denys Mishunov offers an excellent tutorial on both the Intersection Observer and its application for lazy loading images. Here’s how his solution is structured.
Suppose you want to lazy load an image gallery. The markup for each image would be as follows:
<img data-src="image.jpg" alt="test image">
In this example, the image path is stored in a data-src
attribute rather than the src
attribute. This approach prevents the image from loading immediately, which is not the desired behavior.
In the CSS, you can assign a min-height
value to each image, for instance, 100px. This creates a vertical space for the placeholder (the <img>
element without a src
attribute):
img {
min-height: 100px;
/* additional styles here */
}
Next, in your JavaScript file, you’ll need to create a configuration object and register it with an IntersectionObserver
instance:
// create a configuration object: rootMargin and threshold
const config = {
rootMargin: '0px 0px 50px 0px',
threshold: 0
};
// register the configuration object with an instance
const observer = new IntersectionObserver((entries, self) => {
// iterate through each entry
entries.forEach(entry => {
// process only the images that are intersecting
if (entry.isIntersecting) {
// custom function that transfers the path from data-src to src
preloadImage(entry.target);
// stop observing the image as it's now loaded
self.unobserve(entry.target);
}
});
}, config);
Finally, iterate over all your images and add them to the IntersectionObserver
instance:
const imgs = document.querySelectorAll('[data-src]');
imgs.forEach(img => {
observer.observe(img);
});
The advantages of this solution are its simplicity, effectiveness, and the fact that the Intersection Observer handles the heavy lifting regarding calculations.
Regarding browser support, all major browsers support the Intersection Observer API in their latest versions, except for Internet Explorer 11 and Opera Mini.
For more in-depth information about the Intersection Observer API and the specifics of this implementation, check out Denys’s article.
3. Lozad.js
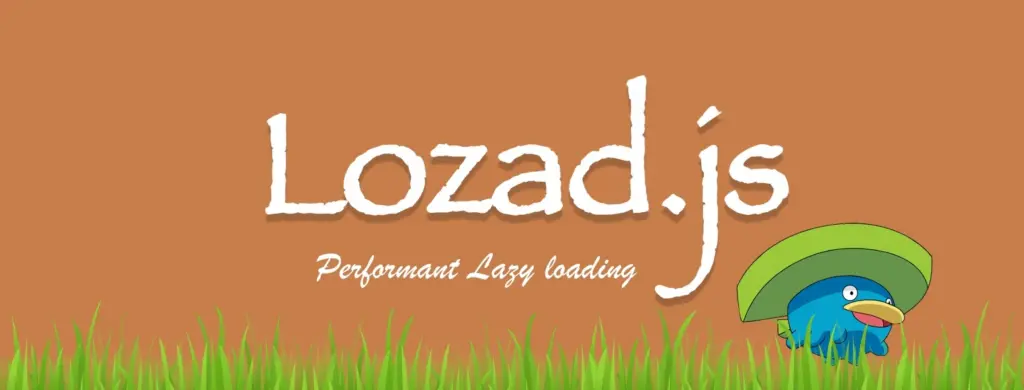
A quick and straightforward alternative for implementing lazy loading of images is to utilize a JavaScript library that handles much of the work for you.
Lozad.js is a lightweight, highly efficient, and configurable lazy loader that relies solely on pure JavaScript and has no external dependencies. It’s an excellent option for lazy loading images, videos, and iframes as users scroll.
You can easily install Lozad using npm or Yarn, and import it using your preferred module bundler:
//npm
npm install --save lozad
//yarn
yarn add lozad
To use it, import it in your JavaScript file:
import lozad from 'lozad';
Alternatively, you can download the library via a CDN and include it at the bottom of your HTML page with a <script>
tag:
<script src="https://cdn.jsdelivr.net/npm/lozad/dist/lozad.min.js"></script>
For a basic implementation, simply add the class lozad
to the image in your markup:
<img class="lozad" data-src="img.jpg">
Then, instantiate Lozad in your JavaScript file:
const observer = lozad();
observer.observe();
You can find comprehensive documentation on using the library in the Lozad GitHub repository.
If you prefer not to dive into the Intersection Observer API or seek a quick implementation that works for various content types, Lozad.js is an excellent choice.
4.Lazy Loading with a Blurred Image Effect
4.Lazy Loading with a Blurred Image Effect
If you’re a regular reader on Medium, you may have observed how the platform loads the primary image in a post. Initially, you see a blurred, low-resolution version of the image while the high-resolution version is being lazy loaded.You can create a similar effect when implementing lazy loading for images in HTML by combining CSS and JavaScript.
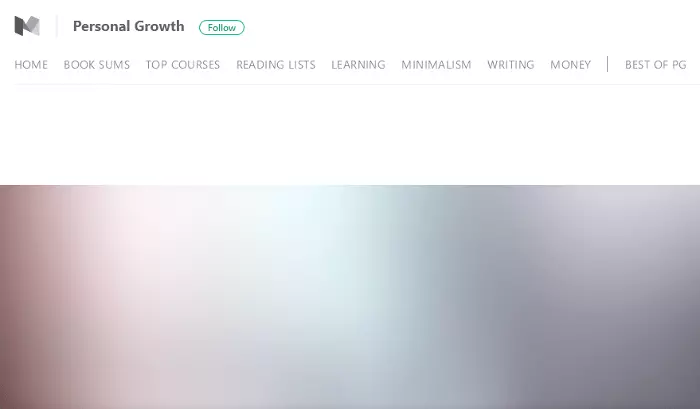
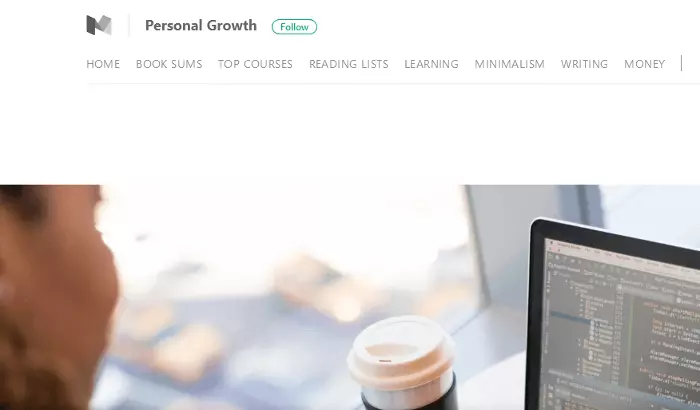
You can implement lazy loading for images with a captivating blurring effect through several methods.
One of my favorite techniques comes from Craig Buckler. Here are the highlights of this solution:
- Performance: It consists of only 463 bytes of CSS and 1,007 bytes of minified JavaScript code.
- Retina Screen Support: It’s optimized for high-resolution displays.
- No Dependencies: This method requires neither jQuery nor any other libraries or frameworks.
- Progressive Enhancement: It’s designed to provide functionality for older browsers and scenarios where JavaScript may fail.
You can find all the details in “How to Build Your Own Progressive Image Loader” and download the code from the project’s GitHub repository.
5. Yall.js
Yall.js is a robust JavaScript library that utilizes the Intersection Observer API for lazy loading content as users scroll. It supports various types of media, including images, videos, and iframes, and seamlessly falls back on traditional event handler techniques when necessary.
To include Yall.js in your document, initialize it with the following code:
<script src="yall.min.js"></script>
<script>
document.addEventListener("DOMContentLoaded", yall);
</script>
For lazy loading a simple image, your markup should look like this:
<img
class="lazy"
src="placeholder.jpg"
data-src="image-to-lazy-load.jpg"
alt="Alternative text to describe image."
>
Key Points:Add the class lazy
to the image element.Use a placeholder image in the src
attribute.Place the path of the image you want to lazy load in the data-src
attribute.
Conclusion
There you have it—five effective methods for lazy loading images that you can start experimenting with in your projects. Mastering lazy loading is an invaluable skill for any web developer. To learn more techniques or share your expertise, consider joining the SitePoint forum on image optimization.
FAQs About Lazy Loading Images
What Is Lazy Loading Images?
Lazy loading is a web development technique that enhances the performance of web pages by deferring the loading of certain elements, such as images, until they are actually needed. This means that images are loaded only when they enter the user’s viewport or become visible on the web page, rather than being loaded immediately when the page is rendered.
What Are the Benefits of Lazy Loading Images?
- Improved Website Performance: Reduces initial loading time and speeds up page rendering.
- Reduced Bandwidth Usage: Loads only necessary images, conserving data.
- Enhanced User Experience: Users can interact with the visible content faster.
- Lower Server Load: Improves overall efficiency by decreasing the demand on the server.
To implement lazy loading in HTML, use the loading
attribute. This standard HTML attribute allows you to control when an image should be loaded. By adding loading="lazy"
to the <img>
element, you instruct the browser to load the image only as it approaches the viewport. Here’s an example:
<img src="image.jpg" alt="Description" loading="lazy">
Is Lazy Loading Images Good?
Yes, lazy loading images is a beneficial practice in web development for several reasons:
- Faster Page Loading Speed: By deferring non-essential image loading, lazy loading enhances initial page rendering.
- Bandwidth Conservation: This approach is advantageous for users with limited data plans or slower internet connections.
- SEO Benefits: Improved page loading speeds positively impact search engine optimization (SEO), as faster loading times are a known ranking factor.
- Progressive Enhancement: Lazy loading aligns with progressive enhancement principles by reducing perceived wait times and providing visual cues during loading.
How Do You Know If an Image Is Lazy Loaded?
To verify that you have implemented lazy loading correctly, inspect the HTML source code or use browser developer tools:
- Right-click on the image and select “Inspect” or “Inspect Element.”
- Check the
<img>
element in the developer tools panel. - Look for the
loading
attribute. If it’s set tolazy
, the image is configured for lazy loading.
How Can I Implement Lazy Loading for Images Using JavaScript?
You can implement lazy loading for images using libraries like Lozad.js or Yall.js, or you can create a custom solution with the Intersection Observer API. These libraries monitor when elements enter the viewport and load them only at that moment.
Does Lazy Loading Images Affect SEO?
Yes, lazy loading images can positively affect SEO. By improving page load times and reducing initial data load, lazy loading helps enhance your website’s ranking in search engine results. Faster page speeds are crucial for search engines like Google, and lazy loading contributes to this by ensuring that images do not hinder overall performance.
What Is the Difference Between Native Lazy Loading and JavaScript-Based Lazy Loading?
- Native Lazy Loading: Relies on the browser’s built-in support for the
loading
attribute in<img>
and<iframe>
tags. It is simple to implement and requires no additional JavaScript. - JavaScript-Based Lazy Loading: Offers more flexibility and customization, typically using libraries like Lozad.js or the Intersection Observer API.
Are There Any Downsides to Lazy Loading Images?
The main downside is that images may load with a slight delay as the user scrolls down the page. Additionally, some older browsers may not support lazy loading.
Can I Lazy Load Images in WordPress?
Yes, you can easily implement lazy loading for images in WordPress. Many themes now support native lazy loading by default. You can also use plugins like “Lazy Load by WP Rocket” or “Smush” to enable lazy loading without any coding.